sparta → projects → mars 폴더에서 시작!
✔ flask 기본 폴더 구조
프로젝트 폴더 안에,
static, templates 폴더, templates > index.html 파일, app.py 파일 생성
✔ 패키지 설치하기 👉 Flask와 DB 연결
[설정] - [프로젝트] - [Python 인터프리터] - [+] - [flask, pymongo, dnspython 패키지 설치]
✅ POST 연습
1. 요청 정보 : URL= /mars, 요청 방식 : POST
2. 클라(ajax) -> 서버(flask) : name, address, size
3. 서버(flask) -> 클라(ajax) : 메시지를 보냄(주문 완료!)
1) 클라이언트와 서버 연결하기
✔ 서버 코드(app.py)
@app.route("/mars", methods=["POST"])
def mars_post():
sample_receive = request.form['sample_give']
print(sample_receive)
return jsonify({'msg': 'POST 연결 완료!'})
✔ 클라이언트 코드(index.html)
function save_order() {
$.ajax({
type: 'POST',
url: '/mars',
data: { sample_give:'데이터전송' },
success: function (response) {
alert(response['msg'])
}
});
}
-----------------------------
<button onclick="save_order()" type="button" class="btn btn-warning mybtn">주문하기</button>
2) 서버부터 만들기
✔ 서버 코드(app.py)
from pymongo import MongoClient
client = MongoClient('mongodb+srv://test:sparta@cluster0.ucyg0qc.mongodb.net/?retryWrites=true&w=majority')
db = client.dbsparta
------------------------------------
@app.route("/mars", methods=["POST"])
def web_mars_post():
name_receive = request.form['name_give']
address_receive = request.form['address_give']
size_receive = request.form['size_give']
doc = {
'name': name_receive,
'address': address_receive,
'size': size_receive
}
db.mars.insert_one(doc)
return jsonify({'msg': '주문 완료!'})
3) 클라이언트 만들기
✔ 클라이언트 코드(index.html)
function save_order() {
let name = $('#name').val()
let address = $('#address').val()
let size = $('#size').val()
$.ajax({
type: 'POST',
url: '/mars',
data: {name_give: name, address_give: address, size_give: size},
success: function (response) {
alert(response['msg'])
window.location.reload()
}
});
}
4) 완성 확인하기
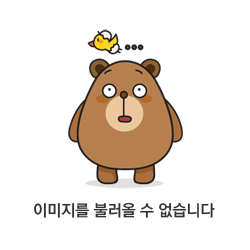
✅ GET 연습
1. 요청 정보 : URL= /mars, 요청 방식 : GET
2. 클라(ajax) -> 서버(flask) : 없음
3. 서버(flask) -> 클라(ajax) : 전체 주문을 보내주기
1) 클라이언트와 서버 연결하기
2) 서버부터 만들기
✔ 서버 코드(app.py)
@app.route("/mars", methods=["GET"])
def web_mars_get():
order_list= list(db.mars.find({}, {'_id': False}))
return jsonify({'orders': order_list})
3) 클라이언트 만들기
✔ 클라이언트 코드(index.html)
<script>
$(document).ready(function () {
show_order();
});
function show_order() {
$.ajax({
type: 'GET',
url: '/mars',
data: {},
success: function (response) {
let rows = response['orders']
for (let i = 0; i < rows.length; i++){
let name = rows[i]['name']
let address = rows[i]['address']
let size = rows[i]['size']
let temp_html = `<tr>
<td>${name}</td>
<td>${address}</td>
<td>${size}</td>
</tr>`
$('#order-box').append(temp_html)
}
}
});
}
4) 완성 확인하기
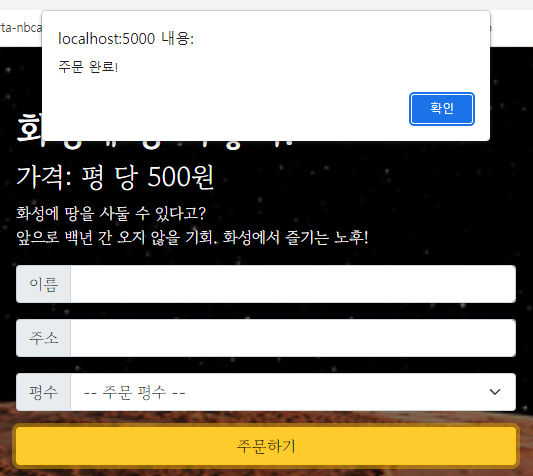
'웹개발 > 수업' 카테고리의 다른 글
웹개발 4주차 개발일지_Sparta Coding Club (0) | 2023.03.02 |
---|---|
웹개발 4주차_스파르타피디아 프로젝트 연습 (0) | 2023.02.27 |
웹개발 3주차 개발일지_Sparta Coding Club (0) | 2023.02.25 |
웹개발 2주차 개발일지_Sparta Coding Club (0) | 2023.02.23 |
웹개발 1주차 개발일지_Sparta Coding Club (0) | 2022.08.25 |