서브루틴 Subroutine
종속적인 루틴
코루틴 Coroutine
협력적으로 실행되는 루틴
파이썬의 코루틴
비동기 프로그래밍
동기 처리 : 순차 처리 방식
비동기 처리 : 여러 작업을 동시에 처리
시간 : 동기 > 비동기
실행 중인 함수를 잠시 중단(대기 상태)하고 나중에 실행을 재생하는 기능
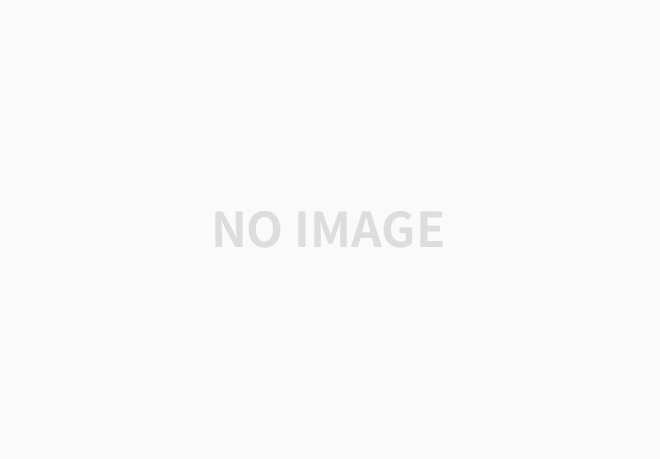
asyncio
import asyncio
import random
# await 코루틴 안에서 다른 코루틴의 실행 완료를 기다림
async def fetch_data():
print("데이터를 가져오는 중...")
await asyncio.sleep(1) # 데이터를 가져오는데 1초가 걸린다고 가정
return random.randint(1, 100)
async def main():
data = await fetch_data()
print(f"가져온 데이터: {data}")
# 코루틴 실행
asyncio.run(main())
coroutine
def my_coroutine():
while True:
value = yield # yield를 만나면 일시 중단
print('Received value:', value)
# 코루틴 객체 생성
co = my_coroutine()
# 코루틴 실행 준비 - iterable한 객체
next(co) # 다음 yield를 만날 때까지 재개
# 값을 보내기
co.send('Hello, world!') # yield의 반환값으로 들어감
###################################
def my_generator():
yield 1
yield 2
yield 3
gen = my_generator()
print(next(gen)) # 1
print(next(gen)) # 2
print(next(gen)) # 3
###################################
def my_coroutine():
while True:
x = yield
print('Received:', x)
co = my_coroutine()
next(co)
co.send(10) # Received: 10
co.send(20) # Received: 20
co.send(30) # Received: 30
###################################
phone_book = {"John": "123-4567", "Jane": "234-5678", "Max": "345-6789"}
def search():
name = yield
while True:
if name in phone_book:
phone_number = phone_book[name]
else:
phone_number = "Cannot find the name in the phone book."
name = yield phone_number
# 코루틴 객체 생성
search_coroution = search()
next(search_coroution)
# 검색 예시
result = search_coroution.send("John")
print(result) # 123-4567
result = search_coroution.send("Jane")
print(result) # 234-5678
result = search_coroution.send("Sarah")
print(result) # Cannot find the name in the phone book.
https://docs.python.org/ko/3/library/asyncio.html
asyncio — Asynchronous I/O
Hello World!: asyncio is a library to write concurrent code using the async/await syntax. asyncio is used as a foundation for multiple Python asynchronous frameworks that provide high-performance n...
docs.python.org
파이썬 코딩 도장: 47.10 asyncio 사용하기 (dojang.io)
파이썬 코딩 도장: 47.10 asyncio 사용하기
asyncio(Asynchronous I/O)는 비동기 프로그래밍을 위한 모듈이며 CPU 작업과 I/O를 병렬로 처리하게 해줍니다. 동기(synchronous) 처리는 특정 작업이 끝나면 다음 작업을 처리하는 순차처리 방식이고, 비동
dojang.io
'Python > 수업' 카테고리의 다른 글
객체지향(Object Oriented Programming) 특강 (1) | 2023.04.28 |
---|---|
파이썬 대패키지시대_패키지 의존성 관리 (6) | 2023.04.23 |
프로세스와 스레드 (2) | 2023.04.16 |
타입별 메서드의 종류 (0) | 2023.04.16 |
파이썬 문법 심화 1주차 3_Sparta Coding Club (0) | 2023.03.23 |